Making cool art with Sass and CSS
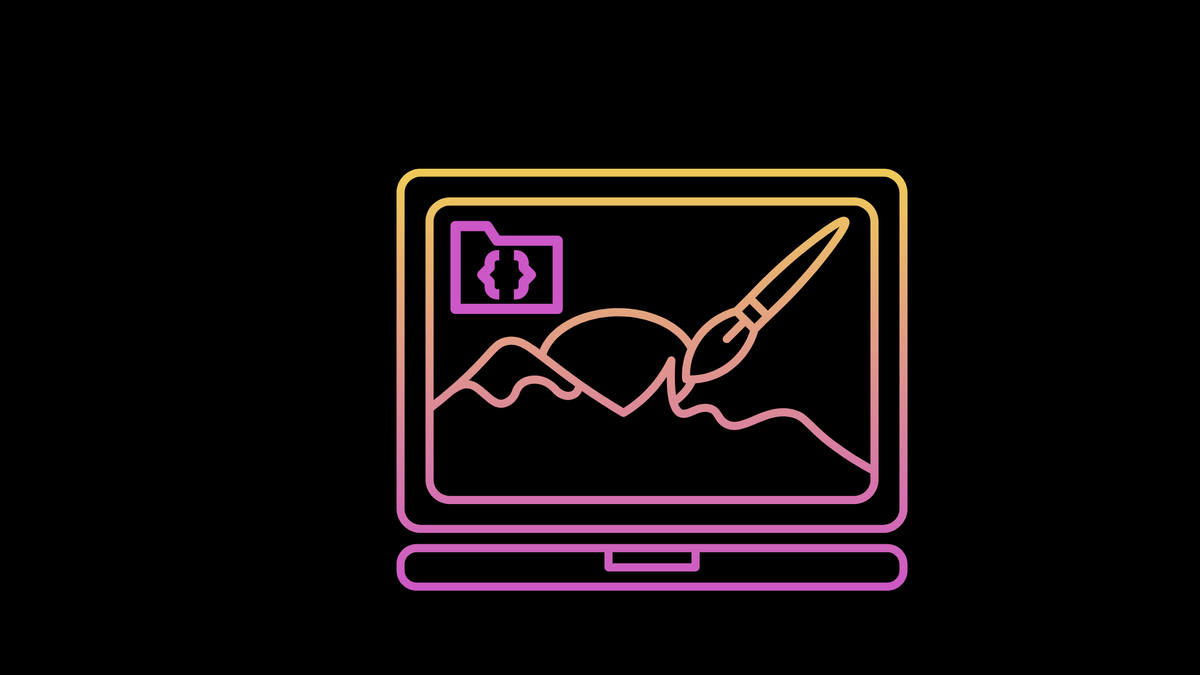
Taking, a break from my more intensive projects I decided to create a small project showcasing front-end skills. This was originally for a previous challenge at the DEV Community to make art using CSS. It has been a while since I have worked with graphic design and programming. To ease my boredom I decide to make a CSS art project.
Gathering references
What am I going to make for my art project? First I had to get an idea and reference that I would use. I wanted to start with a basic design and did not want to do anything complex for a small project. I searched for CSS art to look for examples.
I was inspired by Shunya Koide, a programmer and graphic designer with a vast and impressive portfolio. I was attracted to the Sphere and decided to try make recreate it.
Prerequisites
- a text editor or IDE. I used VSCode
- HTML, CSS, and Sass
- a reference image, if you want to create something different (optional)
Creating the background
In the first part, we create the background. We are recreating the split-screen background. The pyramid is in the center of the screen.
body {
margin: 0;
padding: 0;
width: 100%;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
background: linear-gradient(#a97ee1 54%, #9a71b2 54% 54.2%, #cbcbcb 54.2%);
}
Creating and animating the pyramid
Another part is creating the 3D pyramid shape in the center. The 3d pyramid by Chris Bongers was the reference used to make the pyramid. A pyramid has four sides and a base. In the HTML file create the four sides and the base.
<div class="box">
<link rel="stylesheet" type="text/css" href="style.css" />
<div class="pyramid">
<div class="base"></div>
<div class="side one"></div>
<div class="side two"></div>
<div class="side three"></div>
<div class="side four"></div>
</div>
</div>
Next, style the sides in the CSS file. First, we are going to create a wrapper for the pyramid. After creating each side individually, various purple shades each side of the triangle for the color.
.box {
position: relative;
}
/* wrapper for pyramid */
.pyramid {
position: relative;
width: 200px;
height: 200px;
transform-style: preserve-3d;
transform: rotateY(326deg) rotateX(2deg) rotateZ(359deg);
}
/*pyramid sides
*/
.side {
width: 0;
height: 0;
position: absolute;
opacity: 0.7;
border: 100px solid transparent;
border-bottom: 200px solid #9d2cac;
border-top: 0px;
}
.one {
transform: rotateX(30deg);
transform-origin: 0 0;
}
.two {
transform-origin: 100px 0;
transform: rotateY(90deg) rotateX(-30deg);
border-bottom-color: #a104cb;
}
.three {
transform-origin: 100px 0;
transform: rotateY(270deg) rotateX(-30deg);
border-bottom-color: #8904cb;
}
.four {
transform-origin: 100px 0;
transform: rotateY(0) rotateX(-30deg);
border-bottom-color:#bb33ff;
}
.base {
position: absolute;
width: 100%;
height: 100%;
transform: translateY(73px) rotateX(90deg);
border: 0;
background: #ea65fc;
}
After creating the sides and base, the pyramid will be animated to rotate counter-clockwise.
/* animating the pyramid */
@keyframes rotate {
from {
transform: rotateY(0) rotateX(0deg) rotateZ(0deg);
}
to {
transform: rotateY(360deg) rotateX(0deg) rotateZ(0deg);
}
}
.pyramid {
animation: rotate 5s linear infinite;
}
The final part will be a shadow for the pyramid. The drop-shadow function will create the shadow effect on the pyramid's sides.
filter: drop-shadow(0px 7px 29px rgb(99, 98, 98));
End Result
Here is how to make cool art in HTML and CSS using basic shapes. While it may seem simple, it improves graphic design and programming skills at the same time.